In the past, adding page transitions on web pages has been a simple process. As you click on the link it redirects you to the next page as the browser loads the next page or element. The web has started to feel outdated if you will think about and there is plenty of room to improve. Wouldn’t it be great to add some smooth transitions to create a more proficient user experience the next page loads?
Good thing that it can be done. There are a lot of complicated applications and plugins that can offer the same type of effect and some might think that it’s hard to attain. Luckily, it doesn’t have to be this way.
I’ve been playing around with jQuery to figure out ways to replace the reload of a web page with an awesome animation that will surely blow the mind of the user without the use of plugin. Today I will share with you what I have.
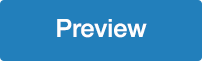
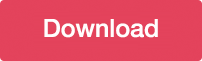
Create the Markup
The HTML structure will just consists of two divs for the two pages which contain main-page and next-page ids.
<div id="main-page"> <!--- Main Content Here --> </div> <div id="next-page"> <!--- Next Page Content Here --> </div>
Let’s add content inside the divs. We will give each page a link to navigate and connect them to each other via mainlink and nextlink class.
<div id="main-page"> <div class="maincontent"> <h1>Full Page Transition</h1> <a class="mainlink">TRY IT NOW ➜</a> </div> </div> <div id="next-page"> <div class="nextcontent"> <h1>Great! You're in the 2nd Page!</h1> <a class="nextlink"> ← GO BACK</a> </div> </div>
Add Style
Now let’s add some general styles on our markup. We will set the whole body element to 100% and set some font properties to our h1 tag.
body { width: 100%; } h1 { font-family: 'Raleway'; font-weight: bold; text-align: center; font-size: 50px; color: #fff; margin-top: 120px; }
Next let’s work on the main-page and next-page styles. Both of them share the same default properties except for the background color. I set the position to absolute and hide both element via display: none.
#main-page { height: 25px; width: 375px; position: absolute; top: 0; bottom: 0; left: 0; right: 0; margin: auto; background-color: #27ae60; display: none; } #next-page { height: 25px; width: 375px; position: absolute; top: 0; bottom: 0; left: 0; right: 0; margin: auto; background-color: #e74c3c; display: none; } .maincontent, .nextcontent { padding-top: 40px; display: none; }
I also added basic styles for the buttons to make them more appealing. I used a border to create a smooth box shape on the buttons and aligned them to the center.
a.back{ font-family: 'Lato'; font-size: 20px; color: #dfdfdf; text-decoration: none; text-align: center; width: 20%; margin: 25px auto 30px auto; display: block; } a.mainlink, a.nextlink { font-family: 'Lato'; color: #fff; border: 3px solid #fff; padding: 15px 10px; display: block; text-align: center; margin: 25px auto; width: 13%; text-decoration: none; cursor: pointer; font-size: 20px; font-weight: 500; } a.mainlink:hover, a.nextlink:hover{ background: #fff; color: #575757; }
Power Up with jQuery
For our jQuery on page load, we will start by creating a rotational function which will perform a custom animation of a set of CSS properties via properties, duration, easing complete values.
$(document).ready(function() { $.fn.animateRotate = function(angle, duration, easing, complete) { var args = $.speed(duration, easing, complete); var step = args.step; return this.each(function(i, e) { args.complete = $.proxy(args.complete, e); args.step = function(now) { $.style(e, 'transform', 'rotate(' + now + 'deg)'); if (step) return step.apply(e, arguments); }; $({deg: 0}).animate({deg: angle}, args); }); };
Next, we will reset all properties of the main page and fade it. When the mainlink and nextlink class gets clicked they will both execute the function above but with different object.
The function will first fade out the text in the main page and makes the object shrink. After the execution the object rotates using a function to transition rotation.
$("#main-page").css("background-color", "#e74c3c"); $("#main-page").css("height", "100vh"); $("#main-page").css("width", "100%"); $("#main-page").fadeIn(); $(".maincontent").fadeIn(); $(".mainlink").on("click", function() { $(".maincontent").fadeOut(); $("#main-page").animate({ width: "25px", height: "375px" }, function() { $(this).animateRotate(90); }); setTimeout(function() { $("#main-page").fadeOut(); }, 1500); setTimeout(function() { $("#next-page").animateRotate(0, 0); $("#next-page").css("height", "25px"); $("#next-page").css("width", "375px"); $("#next-page").fadeIn(); $("#next-page").animate({ backgroundColor: "#27ae60" }, function() { $(this).animate({ height: "100vh" }, function() { $(this).animate({ width: "100%" }, function() { $(".nextcontent").fadeIn(300); }); }); }); }, 800); });
After all of these steps, the function fades out the object that was click on to show the new object and then changes the background color of the object that matches the new object.
Finally, we animate the height and width and fade in the content of the new object displayed.
$(".nextlink").on("click", function() { $(".nextcontent").fadeOut(); $("#next-page").animate({ width: "25px", height: "375px" }, function() { $(this).animateRotate(-90); }); setTimeout(function() { $("#next-page").fadeOut(); }, 1500); setTimeout(function() { $("#main-page").animateRotate(0, 0); $("#main-page").css("height", "25px"); $("#main-page").css("width", "375px"); $("#main-page").fadeIn(); $("#main-page").animate({ height: "100vh" }, function() { $(this).animate({ width: "100%" }, function() { $(".maincontent").fadeIn(300); }); }); }, 1400); }); });
Conclusion
There you have it! The final result is a smooth transition between pages of our site. Finally, elements can now move from one page to another as we fire animations even before it loads the next page.
You can learn more about jQuery animate here.
5 Comments